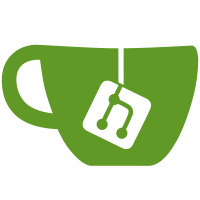
MOVED: * This codebase was originally part of r00t2.io/sysutils but has been split off to its own library.
104 lines
5.3 KiB
Go
104 lines
5.3 KiB
Go
package cryptparse
|
|
|
|
import (
|
|
`crypto`
|
|
`crypto/tls`
|
|
`crypto/x509`
|
|
`encoding/pem`
|
|
`encoding/xml`
|
|
`net/url`
|
|
|
|
`github.com/Luzifer/go-dhparam`
|
|
)
|
|
|
|
// PemBlocks is a combined set of multiple pem.Blocks.
|
|
type PemBlocks []*pem.Block
|
|
|
|
// TlsFlat provides an easy structure to marshal/unmarshal a tls.Config from/to a data structure (JSON, XML, etc.).
|
|
type TlsFlat struct {
|
|
XMLName xml.Name `xml:"tlsConfig" json:"-" yaml:"-" toml:"-"`
|
|
// SniName represents the expected Server Name Indicator's name. See TlsUriParamSni.
|
|
SniName string `json:"sni_name" toml:"SNIName" yaml:"SNI Name" xml:"sniName,attr" required:"true" validate:"required"`
|
|
// SkipVerify, if true, will bypass certificate verification. You generally should not enable this. See TlsUriParamNoVerify.
|
|
SkipVerify bool `json:"skip_verify,omitempty" toml:"SkipVerify,omitempty" yaml:"Skip Verification,omitempty" xml:"skipVerify,attr,omitempty"`
|
|
// Certs contains 0 or more TlsFlatCert certificate definitions. See TlsUriParamCert and TlsUriParamKey as well.
|
|
Certs []*TlsFlatCert `json:"certs,omitempty" toml:"Certs,omitempty" yaml:"Certificates,omitempty" xml:"certs>cert,omitempty"validate:"omitempty,dive"`
|
|
// CaFiles contains filepaths to CA certificates/"trust anchors" in PEM format. They may be combined. See TlsUriParamCa.
|
|
CaFiles []string `json:"ca_files,omitempty" toml:"CaFiles,omitempty" yaml:"CA Files,omitempty" xml:"roots>ca,omitempty" validate:"omitempty,dive,filepath"`
|
|
// CipherSuites represents desired ciphers/cipher suites for this TLS environment. See TlsUriParamCipher.
|
|
CipherSuites []string `json:"cipher_suites,omitempty" toml:"CipherSuites,omitempty" yaml:"Cipher Suites,omitempty" xml:"ciphers,omitempty"`
|
|
// Curves specifies desired cryptographic curves to be used. See TlsUriParamCurve.
|
|
Curves []string `json:"curves,omitempty" xml:"curves>curve,omitempty" yaml:"Curves,omitempty" toml:"Curves,omitempty" validate:"omitempty,dive"`
|
|
// MinTlsProtocol specifies the minimum TLS version. See TlsUriParamMinTls.
|
|
MinTlsProtocol *string `json:"min_tls_protocol,omitempty" xml:"minTlsProtocol,attr,omitempty" yaml:"MinTlsProtocol,omitempty" toml:"MinTlsProtocol,omitempty"`
|
|
// MaxTlsProtocol specifies the maximum TLS version. See TlsUriParamMaxTls.
|
|
MaxTlsProtocol *string `json:"max_tls_protocol,omitempty" xml:"maxTlsProtocol,attr,omitempty" yaml:"MaxTlsProtocol,omitempty" toml:"MaxTlsProtocol,omitempty"`
|
|
}
|
|
|
|
// TlsFlatCert represents a certificate (and, possibly, paired key).
|
|
type TlsFlatCert struct {
|
|
XMLName xml.Name `xml:"cert" json:"-" yaml:"-" toml:"-"`
|
|
// KeyFile is a filepath to a PEM-encoded key file. See TlsUriParamKey.
|
|
KeyFile *string `json:"key,omitempty" xml:"key,attr,omitempty" yaml:"Key,omitempty" toml:"Key,omitempty" validate:"omitempty,filepath"`
|
|
// CertFile is a filepath to a PEM-encoded certificate file. See TlsUriParamCert.
|
|
CertFile string `json:"cert" xml:",chardata" yaml:"Certificate" toml:"Certificate" required:"true" validate:"required,filepath"`
|
|
}
|
|
|
|
// TlsPkiChain contains a whole X.509 PKI chain -- Root CA(s) (trust anchors) which sign Intermediate(s) which sign Certificate(s).
|
|
type TlsPkiChain struct {
|
|
/*
|
|
Roots are all trust anchors/root certificates.
|
|
|
|
Roots are certificates that are self-signed and can issue certificates/sign CSRs.
|
|
*/
|
|
Roots []*x509.Certificate
|
|
// RootsPool is an x509.CertPool representation of Roots.
|
|
RootsPool *x509.CertPool
|
|
/*
|
|
Intermediates are signers that should not be trusted directly, but instead included in the verification/validation chain.
|
|
|
|
Intermediates are certificates that are NOT self-signed (they should be signed by at least one Roots/RootsPool)
|
|
but CAN issue certificates/sign CSRs.
|
|
*/
|
|
Intermediates []*x509.Certificate
|
|
// IntermediatesPool is an x509.CertPool representation of Intermediates.
|
|
IntermediatesPool *x509.CertPool
|
|
/*
|
|
Certificates are "leaf certificates"; typically these are the certificates used directly by servers/users.
|
|
|
|
A certificate is considered a Certificate here if it is NOT self-signed and is NOT able to issue certificates/sign CSRs.
|
|
*/
|
|
Certificates []*tls.Certificate
|
|
// CertificatesPool is an x509.CertPool representation of Certificates.
|
|
CertificatesPool *x509.CertPool
|
|
/*
|
|
UnmatchedCerts contains Certificates that:
|
|
* Do not match any of Roots/RootsPool as its signer, and/or
|
|
* Do not match any Intermediates/IntermediatesPool as its signer, and/or
|
|
* Does not meet requirements for Roots/RootsPool, and/or
|
|
* Does not meet requirements for Intermediates/IntermediatesPool, and/or
|
|
* Has no matching crypto.PrivateKey found.
|
|
|
|
These should generally *never* be used if they were parsed in.
|
|
They represent "stray" certificates that have no logical chain/path found
|
|
and are likely unusable for purposes of this environment.
|
|
*/
|
|
UnmatchedCerts []*x509.Certificate
|
|
// UnmatchedCertsPool is an x509.CertPool representation of UnmatchedCerts.
|
|
UnmatchedCertsPool *x509.CertPool
|
|
/*
|
|
UnmatchedKeys represent parsed private keys that have no matching corresponding certifificate.
|
|
|
|
These should generally *never* be used if they were parsed in.
|
|
They represent "stray" keys that have no logical chain/path found
|
|
and are likely unusable for purposes of this environment.
|
|
*/
|
|
UnmatchedKeys []crypto.PrivateKey
|
|
// DhParams represent any found DH parameters. This will usually be empty.
|
|
DhParams []*dhparam.DH
|
|
}
|
|
|
|
type TlsUri struct {
|
|
*url.URL
|
|
}
|